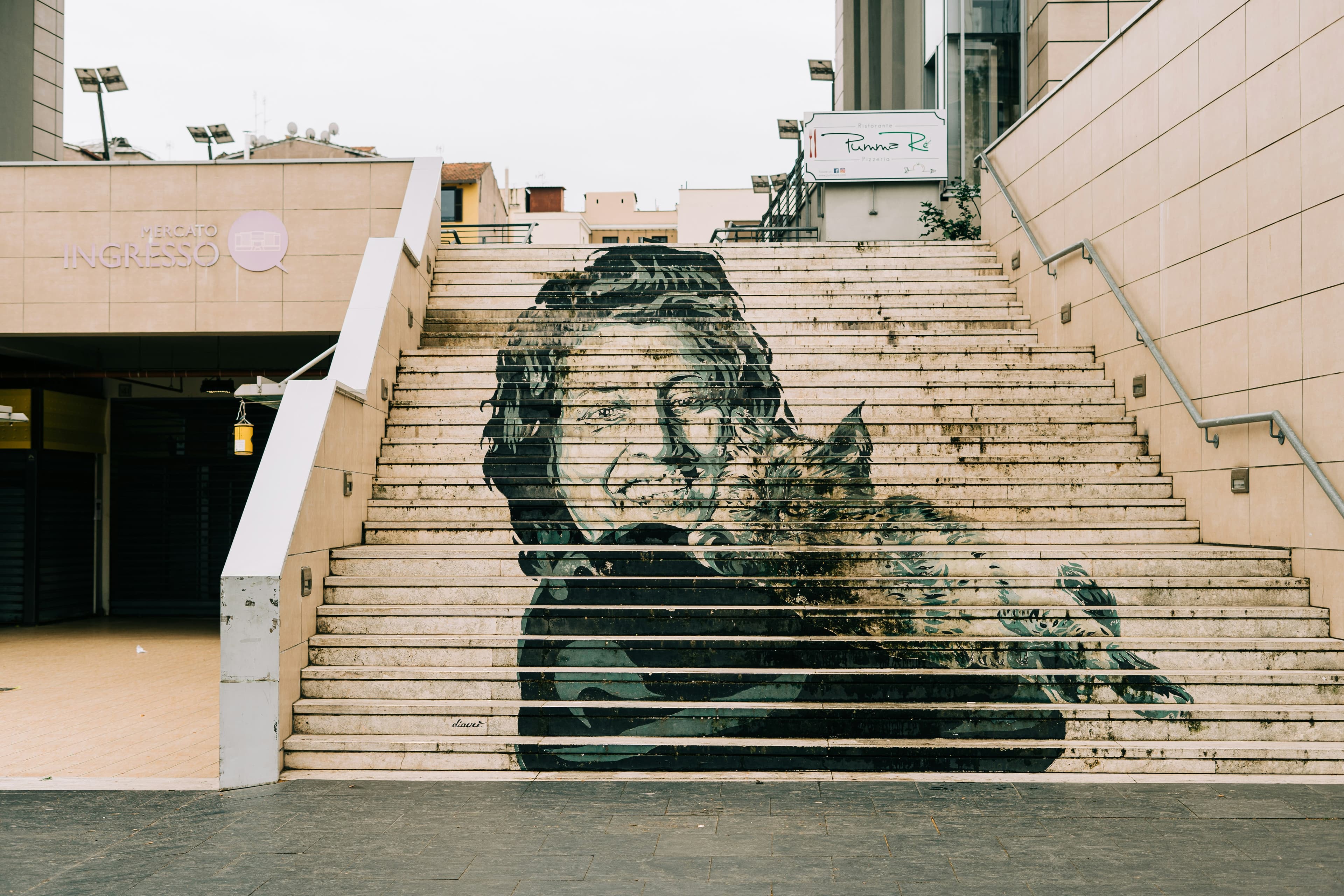
My Approach to solving LeetCode problems
- Authors
- Name
- Stephen ♔ Ó Conchubhair
- Bluesky
- @stethewhitefox.bsky.social
So Why Solve LeetCode Problems ? LeetCode is an online platform for coding interview preparation. By choosing these LeetCode problems, I aim to master coding patterns and interviews, particularly the Big Tech companies (FAANG/MAANG), which heavily focus on data structures and algorithms (DSA). My goal is not only to prepare for these challenging interviews but also to elevate my craft by honing my problem-solving skills and enhancing my approach to technical challenges, as it enables efficient problem-solving.
Adventure in LeetCode/NeetCode Solutions
Join me on an uncomfortable yet educational, and at times fun, adventure into learning problem-solving through solving NeetCode and LeetCode problems. As you read this blog post, feel free at any stage to dive into any of the patterns and explore the example problems associated with them.
Explore Patterns and Examples
If you have any specific questions or need further clarification on any of these patterns, don't hesitate to comment below ! 😊
A Little Break Remember, if you feel overwhelmed at any point, taking a break is always a good idea while working on Twenty eight days leeter part 1, I took a break in Madrid. Sometimes stepping away for a little while can provide a fresh perspective when you return. I hope you enjoy my journey of mastering coding patterns !
For me working on LeetCode problems sharpens both my theoretical knowledge and practical coding skills, making me a more robust and versatile developer.
My Step-by-Step Approach
- Set a Timer:
- For the first try, start with: 40 minutes (Easy), 60 minutes (Medium), 100 minutes (Hard).
- For the second attempt, reduce to 20 minutes (Easy), 40 minutes (Medium), 60 minutes (Hard).
- Target times: 5 - 10 minutes (Easy), 10 - 20 minutes (Medium), 20 - 40 minutes (Hard).
- Understand the Problem:
- Read the problem statement carefully.
- Explain my understanding out loud in plain English to clarify the problem.
- Write and or edit my understanding of the problem in these blog posts.
- Think About Approach:
- Draw (if already drawn review and or edit) a Flow Chart:
- Helps in structuring my thoughts and planning my solution.
- Breaks the problem into smaller parts.
- Add comments to outline my thought process.
- Search for solutions if stuck:
- If bogged down, refer to NeetCode/LeetCode hints/solutions with YouTube and tools like copilot for insights.
- Try a Brute Force Solution:
- Implement a straightforward solution first in pseudocode.
- It may not be the most efficient but helps in understanding the problem better.
- Write Out the Solution Multiple Times:
- Practice coding the solution to improve muscle memory and familiarity.
- Evaluate and Improve: Checking if the solution can be optimized for efficiency (time and space complexity).
- Understand the code that solves the problem.
- Use TypeScript (TS):
- Ensure all solutions are written in TS to maintain consistency and leverage its type safety features. This is particularly useful for my full stack Web App development.
Extra Tips:
- Staying Consistent: Regular practice is key to improvement. Tackling at least one problem every day until I can solve it confidently without help, aiming for target times. Setting target times for solving coding problems is a great way to build efficiency and improve my problem-solving skills under time constraints.
- Review After Solving: Reflect on what went well and where I can improve by writing / editing these blog posts.
- Learn from Mistakes: Analyze where I got stuck and how I resolved it bug fix.
- Utilize Tools: For flow charts I am using miro.new and I am subscribed to YouTube channel NeetCode.
1. Vertical Scanning Solution
To learn the Vertical Scanning Pattern I am choosing "Longest Common Prefix" a common interview question. Mastering this pattern can boost your confidence and performance in technical interviews. I am applying my approach above to solve it.
Let's understand the pattern using the LeetCode problem 14. Longest Common Prefix The task is to find the longest common prefix among an array of strings. For example, given ['flower', 'flow', 'flood']
the longest common prefix is flo
.
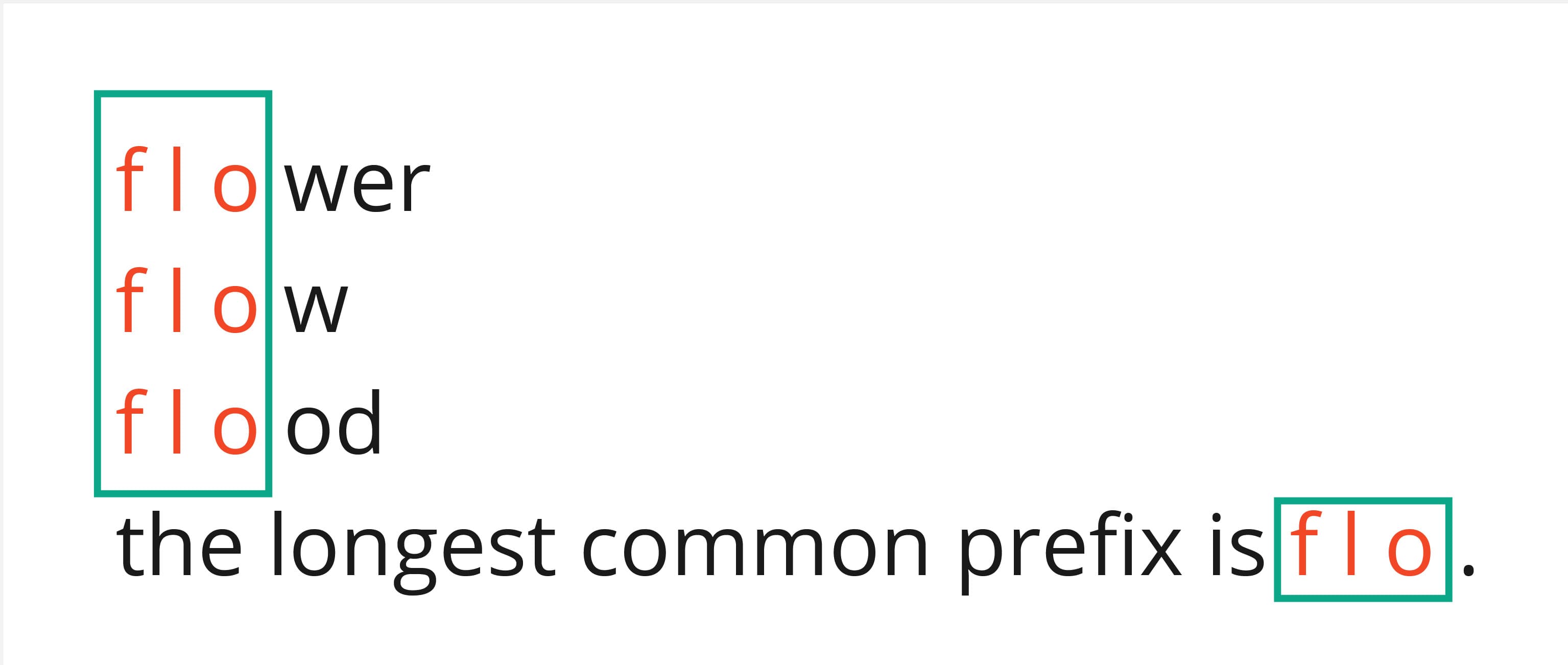
Pseudocode (Brute Force 💪)
// Function to find the longest prefix
function longestCommonPrefix (stringsArr):
// If the input array is empty, return an empty string
if stringsArr.length === 0: return ""
// Assume the first string is the prefix
prefix = stringsArr[0] // prefix is "flower" here
// Loop thru array to find comparisons
for i from 0 to stringsArr.length - 1:
while stringsArr[idx].indexOf(prefix) !== 0: // Check if the current prefix is a prefix of stringsArr[i]
prefix = prefix.substring(0, prefix.length - 1) // Reduce the prefix by one character
if prefix.length === 0: return "" // No common prefix return empty string
// If there is a prefix return it
return prefix
// Example usage
const stringsArr: string[] = ['flower', 'flow', 'flood']
console.log('longestCommonPrefix', longestCommonPrefix(stringsArr)) // 'flo'
TypeScript (Efficient 🎯)
Intuition
The Vertical Scanning pattern involves comparing characters of each string in a vertical manner (character by character across all strings) to find the common prefix.
Step-by-Step Approach:
- Check for edge case: If the input array is empty, return an empty string.
- Character comparisons: Iterate thru the characters of the first string.
- Vertical comparison For each character, compare it character with the character at the same position in all other strings.
- Handle mismatch: Check if we reach the end of one string or find a mismatch, return the substring up to the current index.
- Return result: If we exit the loop, the entire first string is a common prefix.
Complexity
Time: 𝑂(𝑛 ∗ 𝑚) 𝑛 the number of strings and 𝑚 is the length of shortest string in the array
stringsArr
Worst case, each character of the first string (total of 𝑚 characters) is compared with the corresponding character of each of the 𝑛 strings.
Space: 𝑂(1)
// Function to find the longest prefix
const longestCommonPrefix = (stringsArr: string[]): string => {
// If the input array is empty, return an empty string
if (stringsArr.length === 0) return ""
// Iterate thru each character of the first string
for (let idx = 0; idx < stringsArr[0].length; idx++) {
const char = stringsArr[0][idx]
// Compare this character with the character at the same position in all other strings
for (let j = 1; j < stringsArr.length; j++) {
// If we reach the end of one string or find a mismatch
if (idx === stringsArr[j].length || stringsArr[j][idx] !== char) {
return stringsArr[0].substring(0, idx)
}
}
}
// If we exit the loop, the entire first string is a common prefix
return stringsArr[0]
}
// Example usage
const stringsArr: string[] = ["flower", "flow", "flight"];
console.log(longestCommonPrefix(stringsArr)) // Output fl
Photo by Gabriella Clare Marino on Unsplash