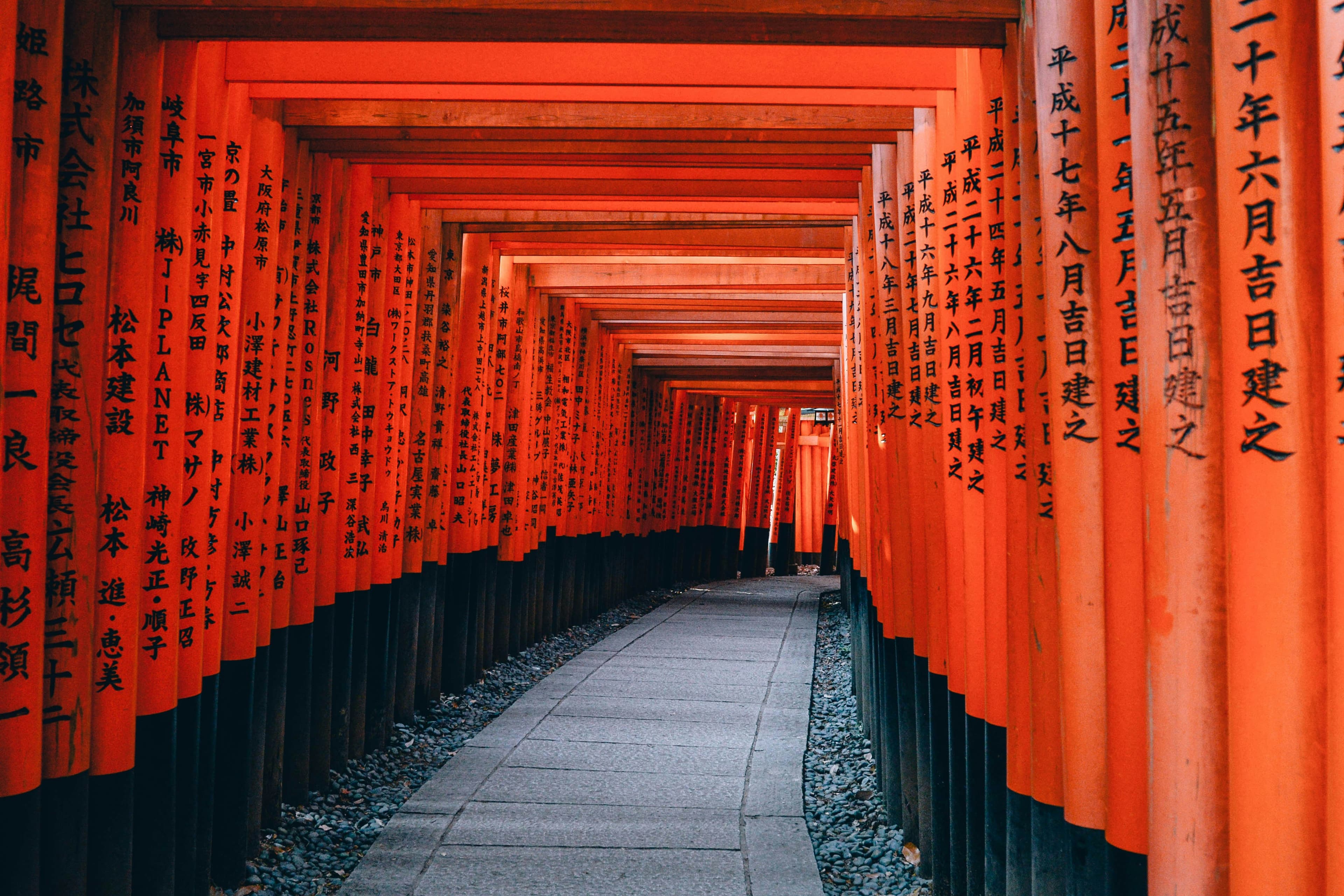
LeetCode: Starting Over NeetCode 150
- Authors
- Name
- Stephen ♔ Ó Conchubhair
- Bluesky
- @stethewhitefox.bsky.social
Introduction
Join me on an adventure of transformation as I navigate the challenging yet rewarding path to mastering problem-solving skills through NeetCode. Whether you're preparing for coding interviews or looking to improve your programming abilities, I believe this structured approach to tackling 150 NeetCode problems is the key to success.
Why Start Over?
After reflecting on my previous attempts, I realized the need for a more organized approach. By categorizing problems and focusing on Data Structures and Algorithms (DSA), I can build a strong foundation and solve challenges more efficiently.
I came to this realization the hard way, while working through 28 Days "Leeter" Part 1 & Part 2 LeetCode problems. This led me to reassess my approach, and I decided to start over. You can read more about my approach solving LeetCode problems.
Categories and Patterns
By categorizing problems into specific problem-solving strategies and algorithms/patterns, it becomes easier to focus on mastering one type of problem at a time. Grouping them in this way leads to a more efficient and effective learning process.
- Arrays & Hashing
- Two Pointers
- Sliding Window
- Stack
- Binary Search
- Backtracking
- Graphs
- Advanced Graphs
- 1-D Dynamic Programming
- 2-D Dynamic Programming
- Greedy
- Intervals
- Math & Geometry
- Bit Manipulation
- Importance of Repetition
- Reference
Arrays & Hashing
First up, Arrays & Hashing, check out my post on Arrays and Hashing with TypeScript for a brief outline of what they are and why we use them. You can also click the links below to go directly to the NeetCode problems and solutions that I have tackled so far.
- Contains Duplicate checks if there are any duplicates in an array.
- Valid Anagram determines if two strings are anagrams.
- Two Sum finds two numbers in an array that add up to a specific target.
- Group Anagrams groups anagrams together in an array of strings.
- Top K Frequent Elements identifies the k most frequent elements in an array.
- Encode and Decode Strings: Encodes a list of strings to a single string and decodes it back to the list.
- Product of Array Except Self: Calculates the product of all elements in the array except the current one for each element.
- Valid Sudoku: Validates whether a given Sudoku board configuration is valid.
Two Pointers
- Valid Palindrome
- Two Sum II
- 3Sum
- Container with Most Water
- Trapping Rain Water
Sliding Window
- Best Time to Buy & Sell Stock
- Longest Substring Without Repeating Characters
- Longest Repeating Character Replacement
- Permutation in String
- Minimum Window Substring
- Sliding Window Maximum
Stack
- Valid Parentheses
- Min Stack
- Evaluate Reverse Polish Notation
- Generate Parentheses
- Daily Temperatures
- Car Fleet
- Largest Rectangle in Histogram
Binary Search
- Search a 2D Matrix
- Koko Eating Bananas
- Search Rotated Sorted Array
- Find Minimum in Rotated Sorted Array
- Time Based Key-Value Store
- Binary Search Find Median of Two Sorted Arrays
Backtracking
- Reverse Linked List
- Merge Two Linked Lists
- Reorder List
- Remove Nth Node from End of List
- Copy List with Random Pointer
- Add Two Numbers
- Linked List Cycle
- Find the Duplicate Number
- LRU Cache
- Merge K Sorted Lists
- Reverse Nodes in K-Group
Graphs
- Number of Islands
- Clone Graph
- Max Area of Island
- Pacific Atlantic Waterflow
- Surrounded Regions
- Rotting Oranges
- Walls and Gates
- Course Schedule
- Course Schedule II
- Redundant Connection
- Number of Connected Components in Graph
- Graph Valid Tree
- Word Ladder
Advanced Graphs
- Reconstruct Itinerary
- Min Cost to Connect all Points
- Network Delay Time
- Swim in Rising Water
- Alien Dictionary
- Cheapest Flights with K Stops
1-D Dynamic Programming
- Climbing Stairs
- Min Cost Climbing Stairs
- House Robber
- House Robber II
- Longest Palindroming Substring
- Palindrome Substrings
- Decode Ways
- Coin Change
- Maximum Product Subarray
- Word Break
- Longest Increasing Subsequence
- Partition Equal Subset Sum
2-D Dynamic Programming
- Unique Paths
- Longest Common Subsequence
- Best Time to Buy/Sell Stock With Cooldown
- Coin Change II
- Target SUm
- Interleaving String
- Longest Increasing Path in a Matrix
- Distinct Subsequences
- Edit Distance
- Burst Balloons
- Regular Expression Matching
Greedy
- Maximum Subarray
- Jump Game
- Jump Game II
- Gas Station
- Hand of Straights
- Merge Triplets to Form Target Triplet
- Partition Labels
- Valid Parenthesis String
Intervals
- Insert Interval
- Merge Intervals
- Non-Overlapping Intervals
- Meeting Rooms
- Meeting Rooms II
- Minimum Interval to Include Each Query
Math & Geometry
- Rotate Image
- Spiral Matrix
- Set Matrix Zeroes
- Happy Number
- Plus One
- Pow(x, n)
- Multiply Strings
- Detect Squares
Bit Manipulation
- Single Number
- Number of 1 Bits
- Counting Bits
- Reverse Bits
- Missing Number
- Sum of Two Integers
- Reverse Integer
Importance of Repetition
As highlighted in the NeeCode video on learning maths, and something that resonated with me (how I certainly learned maths), repetition plays a crucial role in reinforcing concepts and techniques. The same principle applies to learning coding problems. By repeatedly practicing various types of problems, you can achieve the following benefits:
Understanding Patterns: Recognizing common patterns and techniques used in solving problems.
Improving Efficiency: Developing the ability to solve problems more quickly and accurately.
Building Confidence: Gaining confidence in tackling new and complex problems by building a strong foundation.
This structured and repetitive approach ensures a comprehensive understanding and mastery over different types of LeetCode problems.